Vue3-Day2
响应式数据
基础类型的响应式数据(ref)
在setup中,使用ref函数设置响应式数据。
数据会变成对象,在之后的使用中,需要.value
去获取或修改值
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47
| <template> <div class="person"> <h2>name:{{ name }}</h2> <h2>age:{{ age }}</h2> <button @click="changeName">修改名字</button> <button @click="changeAge">修改年龄</button> <button @click="showTel">tel</button> </div>
</template>
<script setup lang="ts" name="Person"> import {ref} from 'vue'
let name = ref('zhangsan') let age = ref(18) let tel = 13812341234
function changeName() { name.value = 'lisi' }
function changeAge() { age.value += 1 }
function showTel() { alert(tel) tel += 1 } </script>
<style> .person { background-color: skyblue; box-shadow: 0 0 10px; border-radius: 10px; padding: 20px; }
button { margin: 0 5px; } </style>
|
对象类型的响应式数据(reactive)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60
| <template> <div class="person"> <h2>brand:{{ car.brand }} ,price:{{ car.price }} mi</h2> <button @click="changePrice">changePrice</button> </div>
<ul> <li v-for="game in games" :key="game.id">{{ game.name }}</li> </ul>
<button @click="changeGame">changeFirstGameName</button> </template>
<script setup lang="ts" name="Person"> import {reactive} from 'vue'
let car = reactive({ brand: 'xiaomi', price: 1000 })
let games = reactive([{ id: 'game1', name: "name1" }, { id: 'game2', name: "name2" }, { id: 'game3', name: "name3" }])
function changePrice() { car.price += 10 }
function changeGame() { games[0].name = 'gameChange' } </script>
<style> .person { background-color: skyblue; box-shadow: 0 0 10px; border-radius: 10px; padding: 20px; }
button { margin: 0 5px; }
li { font-size: 20px; } </style>
|
1 2
| ref:可以定义基本类型和对象类型的响应式数据 reactive:只能定义对象类型的响应式数据
|
1 2 3
| ref包含了reactive, 但是ref必须使用.value,例如ref(xx),需要使用xx的话就需要xx.value,无论xx是什么类型(xx.value.name, xx.value[0]) 可以使用volar插件(包含在Vue-Official中)来提醒每次使用ref时需要添加.value的问题
|
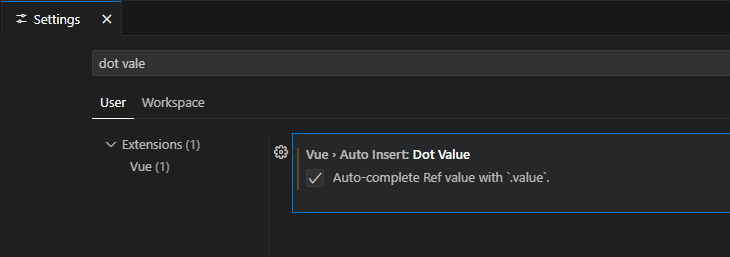
1 2 3 4
| 使用reactive,重新分配一个对象时,会使数据失去响应式,需要使用的话得使用Object.assign(o1, o2) o2的属性都赋值给o1
但是ref可以直接赋值
|
1 2 3 4 5
| <script> function changeCar() { Object.assign(car, {brand: 'audi', price: 888}) } </script>
|
总结
直接使用ref,遇到问题再使用reactive,有经验的自己写
解构响应式数据
toRefs
将数据结构成ref
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| <script setup lang="ts" name="Person"> import {ref, reactive, toRefs} from 'vue'
let person = reactive({ name: 'zhangsan', age: 18 })
let {name, age} = toRefs(person)
function changeName() { person.name += '=' console.log(person.name name.value ) }
function changeName2() { name.value += '=' console.log(name) } </script>
|
toRef
1 2 3 4 5 6 7 8 9 10 11 12 13
| <script setup lang="ts" name="Person">
import {ref, reactive, toRefs, toRef} from 'vue'
let person = reactive({ name: 'zhangsan', age: 18 })
let name = toRef(person, 'name')
console.log(name.value) </script>
|